linebotの練習でつくったオウム返しボットのコード。
<?php
//名前空間のインポート。一番右の非修飾名でインポートされる。
//これがなくてもフルパスで書けばいい
use \LINE\LINEBot\HTTPClient\CurlHTTPClient;
//LINESDKの読み込み
require_once('/var/seneigo_composer/vendor/autoload.php');
$json_string = file_get_contents('php://input');
$json_object = json_decode($json_string);
$httpClient = new CurlHTTPClient('チャネルアクセストークン');
$bot = new \LINE\LINEBot($httpClient, ['channelSecret' => 'チャネルシークレット']);
$header_signature = $_SERVER['HTTP_X_LINE_SIGNATURE'];
//署名を確認して、正当であればリクエストをパースし配列へ
$events = $bot->parseEventRequest($json_string, $header_signature);
//$event = $events[0];
foreach ($events as $event) {
$reply_token = $event->getReplyToken();
$reply_text = $event->getText(); //受信メッセージ
$bot->replyText($reply_token, $reply_text); //返信
} //foreach
以下にかかれているコードはほぼ以下のページのコピー。消えたら困るので保存しておく。
https://heybro21.com/lineapi-howto/
<?php
//LINE MessagingAPI アクセストークン
$accessToken = 'アクセストークン';
//アクション取得
$json_string = file_get_contents('php://input');
$json_object = json_decode($json_string);
//複数event対応
for ($i = 0; $i <= count($json_object->{"events"}) - 1; $i++) {
//アクション判定
if (strcmp($json_object->{"events"}[$i]->{"type"}, "message") == 0) {
//個人チャット受信時
//応答メッセージ
$replyToken = $json_object->{"events"}[$i]->{"replyToken"};
$return_message_text = $json_object->{"events"}[$i]->{"message"}->{"text"};
//レスポンスフォーマット
$response_format_text = [
[
"type" => "text",
"text" => $return_message_text
]
];
//ポストデータ
$post_data = [
"replyToken" => $replyToken,
"messages" => $response_format_text
];
//curl実行
$curl = curl_init("https://api.line.me/v2/bot/message/reply");
$header = array(
'Content-Type: application/json; charser=UTF-8',
'Authorization: Bearer ' . $accessToken
);
curl_setopt($curl, CURLOPT_POST, TRUE);
curl_setopt($curl, CURLOPT_HTTPHEADER, $header);
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($post_data));
curl_setopt($curl, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($curl, CURLOPT_COOKIEJAR, 'cookie');
curl_setopt($curl, CURLOPT_COOKIEFILE, 'tmp');
curl_setopt($curl, CURLOPT_FOLLOWLOCATION, TRUE);
$result = curl_exec($curl);
curl_close($curl);
}
}
このページのもコピペでいけた
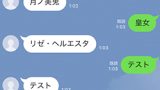
LINEのMessaging APIを使ってみる
LINEのMessaging APIを使って簡単なボットを作成してみます。
アカウント作成
まずはLINE Business IDを作成します。